How to show input fields based on dropdown selected options using jQuery
Hide show input fields based on select value
Many times, while learning a new technology or a framework, it is not sufficient to read just the documentation and syntax, we need to practice. Many of us may try on our own sometimes things will work out for us sometimes we don't know how to evaluate ourselves.
All our recent practice articles were published in that thought of giving readers a practice in jQuery concepts.
All our recent practice articles were published in that thought of giving readers a practice in jQuery concepts.
Follow our FB page @CreativeTechnocrayts
Other jQuery practice articles
1. jQuery DOM functions and array
2. DOM manipulation in jQuery
3. Daily ui practice - Input range sliders
Subscribe
to Creative Technocrayts and get updates in front end development and UI / UX.
Other jQuery practice articles
1. jQuery DOM functions and array
2. DOM manipulation in jQuery
3. Daily ui practice - Input range sliders
Subscribe
to Creative Technocrayts and get updates in front end development and UI / UX.
Some of us might run into a problem of showing input value based on user selecting an option from the dropdown. We can achieve that in many ways.If the user is not selecting a value, the form is incomplete so the user cannot submit the form
To check whether the select value is selected using change function
.change function is used to observe the change in select input.
$("#selectControl").change(function(){
selectedVal = $(this).val();
if(selectedVal == "1"){
// do something..
} else if(selectedVal == "2"){
// do something
} else{
// do something..
}
});
To get the names of the input, which has to be shown or hide:
Pass the id of the inputs set in arrays separately.
var arr1 =['nameFirst', 'ageFirst'];
var arr2 = ['nameSec', 'ageSec'];
How to concatenate two array
.concat is a method used to join two or more arrays and return a new array.
var defaultarr = arr1.concat(arr2);
How to hide / show a value using each function
.val().hide() and .val().show() can be used to show / hide an input.How to check which option is selected from select
$("#selectControl").change(function(){
selectedVal = $(this).val();
if(selectedVal == "1"){
hideFields(arr2);
showFields(arr1);
} else if(selectedVal == "2"){
hideFields(arr1);
showFields(arr2);
} else{
hideFields(defaultarr);
}
});
By checking which value is selected hide fields and show fields function is implemented.
The hide and show fields function take a parameter of array to pass.
Hide and show field inputs using for loop by the names of the input
function hideFields(fields_to_be_hidden) {
var arrayLength = fields_to_be_hidden.length;
for (i=0; i< arrayLength;i++){
var field_required = $('#' + fields_to_be_hidden[i]);
$(field_required).val('').hide();
}
}
function showFields(fields_to_be_shown) {
for (i=0; i< fields_to_be_shown.length;i++){
var field_required = $('#' + fields_to_be_shown[i]);
$(field_required).show();
}
}
Let we have two inputs in the first set and two inputs in the second set. We want the first one to be displayed only when the first input is selected from the selectbox and vice versa.
We pass these two sets of input as array. We iterate through the array, We get the length of the array, until the loop reaches the end, it gets the name of the input. the hide and show of the value is implemented using .hide() and .show() methods in jQuery.
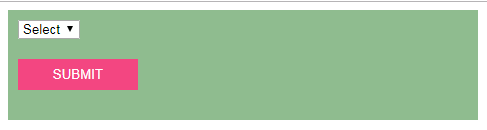
Hide and show input field using .each() function in jQuery
function hideFields(fields_to_be_hidden) {
$.each(fields_to_be_hidden, function(field_id){
var field_required = $('#' + fields_to_be_hidden[field_id]);
$(field_required).val('').hide();
});
}
function showFields(fields_to_be_shown) {
$.each(fields_to_be_shown,function(field_id) {
var field_required_2 = $('#' + fields_to_be_shown[field_id]);
$(field_required_2).val('').show();
});
}
Output while first input is selected
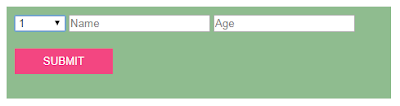
Hide and show input fiels using .map()
function hideFields(fields_to_be_hidden) {
fields_to_be_hidden.map(function(field_id) {
var field_required = $('#' + field_id);
console.log(field_required);
$(field_required).val('').hide();
});
}
function showFields(fields_to_be_shown) {
fields_to_be_shown.map(function(field_id) {
console.log(field_id);
var field_required_2 = $('#' + field_id);
console.log(field_required_2);
$(field_required_2).val('').show();
});
}
CSS for button and form
#submitBtn{
width:120px;
padding:8px 3px;
color:#fff;
text-align:center;
text-transform: uppercase;
background-color:#f34681;
appearance:none;
-webkit-appearance:none;
-moz-appearance:none;
border:0;
box-shadow:none;
margin:20px 0;
}
form{
width: 450px;
background: darkseagreen;
padding: 10px;
}
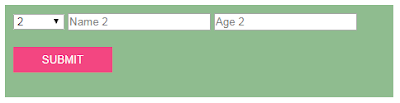
We had seen three ways of implementing solution to show fields based on another select value.
Do contact for colloborating on any of the projects. Glad to work!
Follow our FB page @CreativeTechnocrayts
Comments
Post a Comment