jQuery practice #3 - DOM manipulation
jQuery is a Javascript framework. When do we use Javascript, jQuery, jQuery UI.
a) access parts of a page
b) Modify the apperance of the page
c) Alter the content of the page
d) Respond to users's iteraction with page
e) Add animation to a page
b) .appendTo()
c) .prependTo()
d) .prepend()

Prepending an element using jQuery
New DOM
After the element is append, the HTML looks like,

b) .insertAfter()
c) .before()
d) .insertBefore()
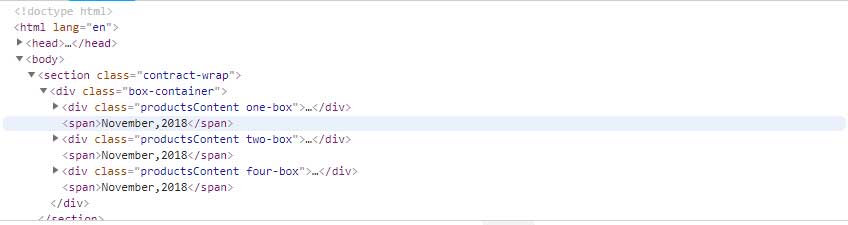
Now, the productsContent class will be wrapped inside .bg-grey class.
b) .text()
Passing a span tag:

.empty() is applied to the paragraph tag inside .productsContent class. The result is,
How to resolve common jQuery errors?
The text inside the p tag is removed, and the tag remains as it is.
Unlike, empty() the remove() function removes the paragraph element itself.So the output looks like,
Subscribe to Creative Technocrayts and be a part of growing community.
DOM Manipulation in jQuery
What jQuery does?
jQuery is a javascript library,a) access parts of a page
b) Modify the apperance of the page
c) Alter the content of the page
d) Respond to users's iteraction with page
e) Add animation to a page
Manipulating DOM with jQuery methods
1. To insert new elements inside every method elements, use
a) .append()b) .appendTo()
c) .prependTo()
d) .prepend()
Example for .prepend() in jQuery
HTML
<div class="productsContent one-box">
<div class="box">
<h4> Loreum ipsum1</h4>
<p> Neque porro quisquam est qui dolorem ipsum quia dolor sit amet,
consectetur, adipisci velit... </p>
</div>
</div>

Prepending an element using jQuery
$('.productsContent').prepend('November,2018');
<div class="productsContent one-box"><span>November,2018</span> <div class="box"> <h4> Loreum ipsum1</h4> <p> Neque porro quisquam est qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit... </p> </div> </div>
Example for .append() in jQuery
$('.productsContent').append('November,2018');
<div class="productsContent one-box"> <div class="box"> <h4> Loreum ipsum1</h4> <p> Neque porro quisquam est qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit... >/p< </div> <span>November,2018</span> </div>

To insert new elements adjacent to every matched elements use:
a) .after()b) .insertAfter()
c) .before()
d) .insertBefore()
Example for .after() in jQuery
$('.productsContent').after('November,2018');
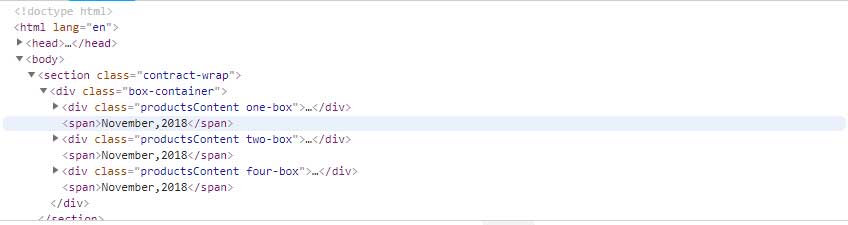
To insert new elements around every matched elements, use
a) .wrap()$('.productsContent').wrap('.bg-grey');
<div class="bg-grey"> <div class="productsContent one-box"> <div class="box"> <h4> Loreum ipsum1</h4> <p> Neque porro quisquam est qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit... >/p< </div> <span>November,2018</span> </div> </div>
To replace every matched element with new elements(s) or text, use
a) .html()b) .text()
$('.productsContent p').html('We are learning jquery!');
$('.productsContent p').html(We are learning JQuery DOM Manipulation');

To remove element(s) inside every matched element, use
a) .empty()$('.productsContent p').empty();
<div class="productsContent one-box"> <div class="box"> <h4> Loreum ipsum1</h4> <p> </p> </div> </div>
The text inside the p tag is removed, and the tag remains as it is.
To remove every matched element in the DOM and descendants from the document without actually deleting them
a) .remove()$('.productsContent p').remove();
<div class="productsContent one-box"> <div class="box"> <h4> Loreum ipsum1</h4> </div> </div>
Other UI practice series:
UI practice exercise - Part 2
jQuery practice - DOM and array functions
Follow our FB page @CreativeTechnocraytsSubscribe to Creative Technocrayts and be a part of growing community.
Comments
Post a Comment